Understanding API Call Timeout Issues in Android Automation
When developing Android applications that interact with external services, API call timeouts can be particularly frustrating roadblocks. These timeouts occur when a request takes longer than the allocated time to complete, resulting in failed operations and poor user experiences. For Android developers working with automation frameworks like Postman, managing these timeout scenarios efficiently is crucial for creating robust applications. As we explore in our conversational AI for business solutions, proper request handling forms the backbone of reliable system interactions, especially when dealing with time-sensitive operations in mobile environments.
The Anatomy of API Timeout Configurations in Postman
Postman, a widely adopted API development environment, offers several timeout configuration options that Android developers should understand thoroughly. At its core, Postman allows you to set both connection timeouts (time allowed to establish a connection) and request timeouts (maximum time allowed for the entire request to complete). According to the official Postman documentation, these settings can be configured globally or per-request basis. When automating API calls for Android applications, particularly those that interface with AI phone systems, carefully calibrating these timeouts based on expected response times and network conditions becomes essential for preventing disruptions during automated test executions.
Implementing Effective Timeout Strategies with Newman CLI
The Newman command-line tool, Postman’s automation companion, provides powerful capabilities for running collections programmatically. When integrating with Android automation workflows, Newman offers specific timeout parameters that can be adjusted through command arguments. For instance, using the --timeout-request
option allows you to set the maximum time (in milliseconds) a request can take. A practical implementation might look like:
newman run your_collection.json --timeout-request 5000
This approach is particularly valuable when creating automated test pipelines for Android applications that communicate with conversational AI platforms, where consistent response timing is critical for maintaining conversation flow integrity.
Android-Specific Solutions for Handling API Timeouts
The Android ecosystem offers several native approaches to handling API timeouts effectively within application code. Using OkHttp, a popular HTTP client for Android, developers can implement timeout controls with remarkable precision. For example:
OkHttpClient client = new OkHttpClient.Builder()
.connectTimeout(15, TimeUnit.SECONDS)
.readTimeout(30, TimeUnit.SECONDS)
.writeTimeout(30, TimeUnit.SECONDS)
.build();
This granular control becomes particularly important when developing applications that interact with AI call center solutions, where maintaining consistent connection parameters ensures reliable communication with backend services during automated testing scenarios.
Automating Postman Collections with Timeout Guards
Creating a bulletproof automation script that handles timeouts gracefully requires implementing robust error handling mechanisms. When automating Postman collections for Android testing, incorporating timeout retry logic can significantly improve test reliability. Tools like Postman’s built-in test scripts allow you to capture timeout failures and implement conditional retry mechanisms. This approach proves especially valuable when testing integrations with AI assistants that may occasionally experience processing delays due to complex conversational analysis.
Leveraging Environment Variables for Dynamic Timeout Management
One of the most powerful aspects of Postman automation is the ability to use environment variables for dynamic configuration. For Android testing workflows, creating environment-specific timeout settings allows for flexible adaptation across development, staging, and production environments. By setting variables like {{connectionTimeout}}
and {{requestTimeout}}
in your Postman environments, you can dynamically adjust these parameters without modifying your collection scripts. This pattern is especially useful when testing Android applications that integrate with white-label AI voice solutions, where environmental differences might necessitate different timeout thresholds.
Monitoring and Analyzing API Timeout Patterns
Effective timeout management requires not just implementation but also ongoing monitoring and analysis. Incorporating logging mechanisms within your Android automation scripts can help identify patterns in API timeout occurrences. Tools like Postman Monitors can be configured to run your collection at regular intervals and report on response times and failures. This data becomes invaluable when optimizing performance for Android applications that leverage SIP trunking services or other communication infrastructure where network latency plays a significant role in timeout frequency.
Advanced Timeout Handling with Custom Newman Reporters
Newman supports custom reporters that can transform test results into actionable insights. For Android automation pipelines, implementing a specialized timeout reporter can provide targeted visibility into timeout-related failures. By focusing specifically on timing-related issues, development teams can quickly identify problematic endpoints or network conditions. This level of insight is particularly valuable when developing Android applications that rely on AI calling services, where distinguishing between normal processing delays and actual timeout problems is crucial for proper system tuning.
Synchronizing Timeout Settings Across Development Teams
Consistency in timeout settings across development environments is essential for reliable testing and production behavior. Creating a centralized configuration repository for timeout settings ensures that all team members and automated processes use identical parameters. Tools like Git can be used to version-control these settings, while CI/CD pipelines can automatically apply them during testing. This coordinated approach is especially important when multiple teams are working on Android applications that interface with complex AI receptionist systems, where inconsistent timeout settings could lead to misleading test results.
Creating Custom Postman Pre-request Scripts for Timeout Intelligence
Postman’s pre-request script functionality offers opportunities for implementing intelligent timeout strategies based on endpoint characteristics. For Android automation, these scripts can dynamically adjust timeout settings based on historical response time data or endpoint complexity. For example:
// Pre-request Script
if (pm.request.url.toString().includes("/large-data-endpoint")) {
pm.request.timeout = 10000; // 10 seconds for data-heavy endpoints
} else {
pm.request.timeout = 3000; // 3 seconds for standard endpoints
}
This adaptive approach is particularly useful when testing Android applications that interact with AI voice agents where response times may vary significantly depending on the complexity of the user query being processed.
Network Condition Simulation for Realistic Timeout Testing
Thorough Android automation testing should include scenarios that simulate various network conditions, as these directly impact timeout behavior. Tools like Charles Proxy or built-in operating system throttling capabilities can simulate slow connections, packet loss, and other challenging network environments. This real-world testing approach helps ensure your handling of timeouts remains robust across the diverse network conditions that mobile users encounter when using applications powered by AI call assistants.
Implementing Progressive Timeout Strategies
Rather than using fixed timeout values, consider implementing progressive timeout strategies that adapt to observed patterns. This approach starts with conservative timeout settings and gradually adjusts based on actual response times. For Android automation frameworks, this can be implemented through custom collectors that track historical performance data and adjust expectations accordingly. This adaptive methodology is especially effective for applications using call center voice AI, where learning from interaction patterns can help optimize timeout parameters for more reliable operation.
Documentation and Communication Protocols for Timeout Management
Clear documentation of timeout strategies and ongoing communication about changes are essential components of effective API management. Creating comprehensive guidelines for timeout handling, including decision trees for different types of failure scenarios, ensures consistent implementation across teams. This aspect becomes particularly important when developing Android applications that integrate with Twilio-based AI solutions, where cross-team coordination between mobile developers and backend service providers requires shared understanding of timeout protocols.
Batch Processing Approaches to Minimize Timeout Impacts
For Android applications dealing with large volumes of API operations, implementing batch processing can significantly reduce timeout-related failures. By grouping related requests and implementing proper sequencing, you can minimize the impact of individual timeouts on overall system operation. This approach pairs well with AI appointment setters and similar solutions where multiple related data operations might be necessary to complete a single business function.
Timeout Strategies for Different API Authentication Methods
Authentication mechanisms directly impact request timing and therefore timeout behavior. OAuth flows, API keys, and JWT tokens each introduce different timing considerations that must be factored into timeout strategies. For Android automation, implementing specific timeout handling for authentication-related operations can prevent cascading failures when initial authentication steps experience delays. This consideration is particularly relevant for applications interfacing with white-label AI sales solutions where secure authentication is combined with complex business logic processing.
Performance Optimization to Reduce Timeout Frequency
Sometimes the best way to handle timeouts is to prevent them entirely through performance optimization. For Android applications, implementing efficient request batching, payload compression, and connection pooling can significantly reduce response times and minimize timeout occurrences. According to web performance research, reducing payload sizes can dramatically improve response times across varying network conditions. These optimization techniques are especially valuable when working with AI sales call platforms where minimizing latency directly impacts conversation naturalness.
Error Reporting and User Experience During Timeout Scenarios
From a user experience perspective, how timeout errors are communicated significantly impacts perceived application quality. Implementing graceful degradation patterns, informative error messages, and automatic retry options (when appropriate) ensures that users aren’t left wondering what happened when timeouts occur. This attention to error handling design is critical for Android applications powered by AI phone services, where maintaining conversation continuity despite occasional network hiccups preserves the natural interaction flow.
Security Implications of Timeout Configuration
Timeout settings aren’t just about user experience—they also have security implications. Excessively long timeouts can potentially leave connections vulnerable to certain types of attacks, while overly aggressive timeouts might trigger unnecessary retry logic that amplifies traffic. According to the OWASP API Security Project, properly configured timeouts are an important aspect of API security posture. This balance is particularly important when developing Android applications that handle sensitive information through conversational AI interfaces.
Testing Frameworks for Timeout Scenario Verification
Comprehensive testing of timeout handling requires specialized approaches. JUnit and Espresso, popular testing frameworks for Android, can be extended with custom rules that simulate timeout conditions and verify proper application behavior. These frameworks allow you to programmatically create challenging network conditions and validate that your application responds appropriately, essential when developing robust Android applications that rely on AI phone numbers for customer communication.
Timeout Optimization Through Continuous Improvement
Timeout management isn’t a one-time configuration task but rather a continuous improvement process. Establishing regular review cycles for timeout-related metrics, failed request analysis, and configuration adjustments ensures that your strategy evolves alongside changing network conditions and user expectations. This approach aligns perfectly with the development methodology for AI call center platforms, where ongoing optimization based on real-world performance data drives continuous system improvement.
Elevate Your Android Communication Stack with Callin.io
If you’re looking to streamline communication processes in your Android applications while addressing API timeout challenges, Callin.io offers a comprehensive solution. Our platform allows you to implement AI-powered phone agents that handle incoming and outgoing calls autonomously, effectively removing many of the timeout concerns that plague traditional API-based communication systems. With natural-sounding voice interactions, our technology can automate appointments, answer common questions, and even close sales while maintaining human-like conversation flow.
Callin.io’s free account gives you access to an intuitive interface for setting up your AI agent, with test calls included and access to the task dashboard for monitoring interactions. For developers seeking advanced capabilities like Google Calendar integration and built-in CRM functionality, our subscription plans start at just 30USD per month. By incorporating Callin.io’s communication stack into your Android applications, you can focus on creating exceptional user experiences rather than managing complex timeout scenarios. Discover more about Callin.io and transform how your applications handle communication today.
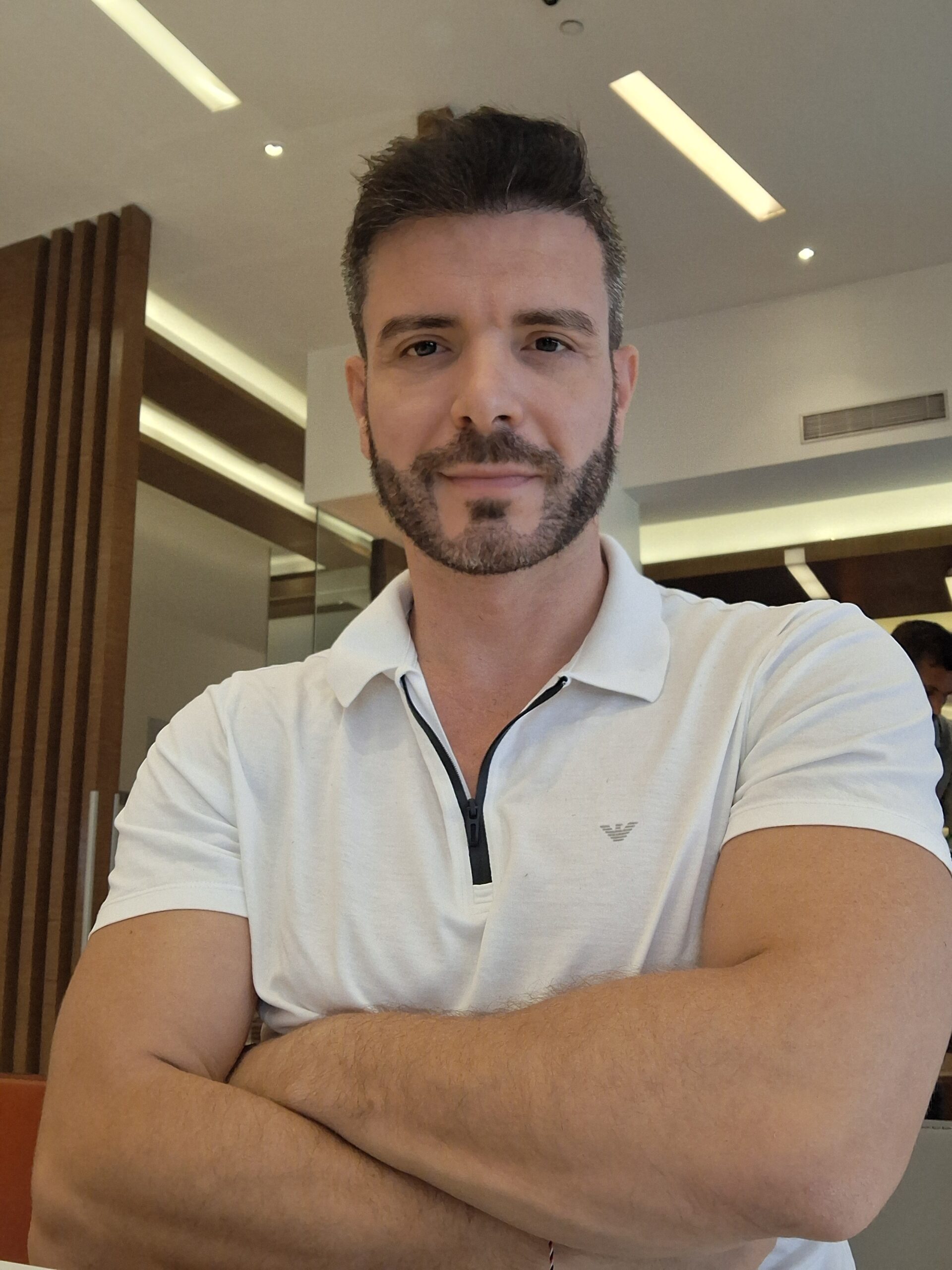
Helping businesses grow faster with AI. 🚀 At Callin.io, we make it easy for companies close more deals, engage customers more effectively, and scale their growth with smart AI voice assistants. Ready to transform your business with AI? 📅 Let’s talk!
Vincenzo Piccolo
Chief Executive Officer and Co Founder